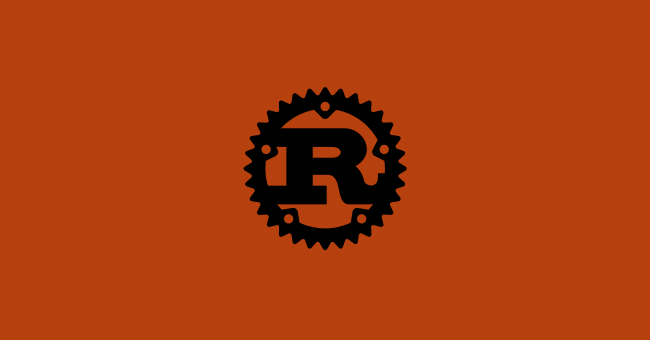
Rust
The core features of rust are:
- Performance
- Reliability
- Productivity
Learning resources
As always, I will leave here my 2 cents in the crazy big book called the world wild web.
- No Boilerplate has an amazing playlist explaining concepts from the beginning and getting you excited to learn rust. You can’t stop binge watching!
- This other post by Brandon Smith talks about strings in rust. For devs coming from every popular languages except C or C++,
strings
are easy to work with. By comparison, strings in rust are a tough cookie. - Let’s get Rust did an amazing video on string types. I recommend it if you have doubts!.
- The book has a good summary on collections (vectors, lists, maps, etc)
- A good alternative to the Rust Book is the Learning Rust page.
- If you want to start with tokio (and you should, because it’s used everywhere) you can peek at this recommendations
- For idioms, design patterns, and anti-patterns it’s good to check this book by the
rust-unofficial
organization.
really good cheatsheets
- The best cheatsheet I found on the web.
How do I learn rust?
I saw the entire playlist of No Boilerplate, just get my feet warm and then with codewars, start to resolve simple katas and learn as I go.
If you are curious about my advance. Here is the repository on github.
tips and tricks in rust
- You need to write code that won’t be used in a short time and don’t like to recieve warnings? write this at the top of your program:
#![allow(unused)]
- There is a macro to sign work in progress in your code, without breaking the complier (like a
pass
equivalent from Python).
todo!()
source: Let’s get rusty - Prototyping in Rust with the todo!() macro
-
You can have an
Enum
with an implementation for just one (or some) of the types. You’ll have to usetraits
andimpl
on your enum.source (and explanation): StackOverflow
-
you can include a static string from a file with
include_string!("file.txt")
source: rust std docs
Inline hints
You can enable and disable suggestions and inline hints in VS Code.
source:Toggle your Rust Analyzer Inlay Hints
Databases
- This video from Let’s get Rusty does an amazing job on Rust databases, please watch it before starting your project if you have the minimal need of a db.
As a sumup table, I have found these packages of interest
package | type | async | connection pool |
---|---|---|---|
rusqlite | driver | ❌ | ❌ |
redis | driver | ❌ | ❌ |
mongodb | driver | ❌ | ❌ |
mysql_common | driver | ❌ | ❌ |
mysql_async | driver | ✅ | ❌ |
scylla | driver | ❌ | ❌ |
postgres | driver | ❌ | ❌ |
sled | driver | ✅ | ❌ |
sqlx | driver | ✅ | ✅ |
diesel | ORM | ❌ | ❌ |
sea-orm | ORM | ✅ | ✅ |
sea-query | query builder | ✅ | ❌ |
r2d2 | connection pool | ✅ | ✅ |
testing
If you want to feel confident of your product, you need to test it.
- Jorge does a great job at explaining testing in rust. Also gives a couple of good tips for advanced users!
- next-test is a better
cargo test
than the default. Try it and see what it looks like. - add coverage with
cargo grcov
.
cargo
section
Cargo is the package manager and a lot more in rust. Here is a tldr on how to use it
cargo build # to build the project
cargo run # to run the project. It builds it if it's not built yet
cargo check # is a great form to call the compiler to check your code
cargo build --release # to build the project with a lot of optimizations
How rustup manages versions and what is a toolchain
As I read this rustup
book, it explains a couple of things about rustup
- it can manage rust
channels
(versions of rust) likestable or beta or nightly
. (Same as NodeJSnvm
or Pythonpyenv
) - you can add or specify
targets
(architectures) like linux-64x, mac, windows, etc. - components are like
tools
that rustup installs. Here arecargo
,rust-analyzer
,clippy
and so on.
linters
clippy
is your friend
clippy
is a linter to catch common errors and mistakes in rust (kinda like flake8
in python).
You can enable categories or enable simple lints to check your code. See the complete list of lints here
TODO:
I'd be nice if I can make 2 configs of clippy:
- one for general fixing, looking for broad fixes
- and one for detailing and setup for production (delete all errors allowance, skips, etc)
bacon
crate is just amazing
bacon
crate is a hot-reload checker for rust with incredible defaults. If you want hot-reload, try bacon
first, and then cargo-watch
.
source: No Boilerplate - Build your lightsaber
Rust hot-reload is available with the crate cargo-watch
You have to install it! Install cargo-watch with
cargo install cargo-watch
and watch your changes on your rust code with:
cargo watch --exec run
to have simply hot reload, and if you want the quieter version:
cargo watch --quiet --clear --exec 'run --quiet'
bonus tip
If you are currently in a parent
folder with many sub projects, you can run a folder like this:
parent/
|-sub_project/
| |-hello.rs
|-sub_project2/
|-other_file.rs
cargo watch --quiet --clear --exec 'run --quiet --subproject hello'
source: Jeremy Chrone - Quiet hot reload with cargo watch -q
To check your dependencies, you can use cargo-outdated
If you see the readme of cargo-outdated
you can see this when you run
cargo outdated
Name Project Compat Latest Kind Platform
---- ------- ------ ------ ---- --------
clap 2.20.0 2.20.5 2.26.0 Normal ---
clap->bitflags 0.7.0 --- 0.9.1 Normal ---
clap->libc 0.2.18 0.2.29 Removed Normal ---
clap->term_size 0.2.1 0.2.3 0.3.0 Normal ---
clap->vec_map 0.6.0 --- 0.8.0 Normal ---
num_cpus 1.6.0 --- 1.6.2 Development ---
num_cpus->libc 0.2.18 0.2.29 0.2.29 Normal ---
pkg-config 0.3.8 0.3.9 0.3.9 Build ---
term 0.4.5 --- 0.4.6 Normal ---
term_size->libc 0.2.18 0.2.29 0.2.29 Normal cfg(not(target_os = "windows"))
Which is nice to have.
do you want to sync the docstring with the README.md of the project?
Try cargo-readme
Look it working here
problems I came across at some point
If you see this problem while compiling a new library on the terminal:
error occurred: Failed to find tool. Is `gcc.exe` installed? (see https://github.com/alexcrichton/cc-rs#compile-time-requirements for help)
The best guide out there is a StackOverflow answer here
some language features
- there are something similar to PEPs in python, but in Rust. They are called RFCs - requests for comments
- it has its own forum where many brilliant discussions are formed
This post comes from github, view it here