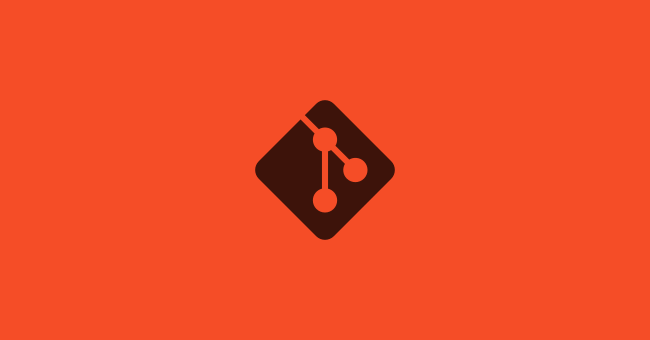
git
git
is such an amazing piece of software. It has some complexity to understand it, but once it clicks, you cannot live without it.
Learning resources
The best tutorial I found of git is the playlist Git and Github for poets - The Coding Train. For real, git is normally not explained clearly to beginners and Daniel Shiffman does a great job here.
Also, there are a couple sites that worth checking:
- This response of Stackoverflow is clear and concise.
- An excellent git cheatsheet per area.
- Which has also a decision tree on how to resolve a git mess.
- Bitbucket has a good guide on how to resolve merge conflicts.
- This article has a nice longer explanation.
- This explains what is
origin
- A more advanced resource, the FreeCAD wiki has a good overview about the source code management.
Using git
You should use standard git commit messages
Like this:
$ git log --oneline -5 --author pwebb --before "Sat Aug 30 2014"
5ba3db6 Fix failing CompositePropertySourceTests
84564a0 Rework @PropertySource early parsing logic
e142fd1 Add tests for ImportSelector meta-data
887815f Update docbook dependency and generate epub
ac8326d Polish mockito usage
This is a nice article explaining the why.
This is a blog post explaining the how.
If you want a reminder, you can check this GitHub Gist about setting up a .gitmessage
file.
clean the merged branches from your local
For POSIX compliant OSes
git branch -d $(git branch --merged=master | grep -v master)
git fetch ---prune
source: this medium post
if you have nushell
shell installed
git branch --merged | lines | where $it !~ '\*' | str trim | where $it != 'master' and $it != 'main' | each { |it| git branch -d $it }
source: this script
delete a branch locally and in the remote
// delete branch locally
git branch -d localBranchName
// delete branch remotely
git push origin --delete remoteBranchName
instead you want to delete a file which is currently tracked?
Of course you can do
rm .\this-file.txt
# and then
git add .\this-file.txt
but
git rm .\this-file.txt --cached
does the same.
The --cached
part does delete the file from the index (adding it to staging area of the next commit), but doesn’t delete the file on your computer.
Keep the file on the repo, but don’t track newer changes
That’s git update-index –skip-worktree file1.txt
is for
you can see the differences with a specific commit
git diff 5eba8a
create a new branch and switch to it
instead of doing
git branch -b newFeature
git switch newFeature
you can do
git checkout newFeature
branches
git branch -m oldName newName # rename a branch
git branch -d goodbye_branch # delete a branch locally
git push origin --delete goodbye_branch # delete a remote branch
git branch # list local branches
git branch -a # list all branches (local and remote)
did you make changes in main?
Don’t worry, git stash
is here to rescue
git stash
git checkout correctBranch
git checkout -b ifYouWantToCreateANewBranch
git stash pop
If you don’t want the stash anymore, you can git stash drop
to delete the topmost stash.
do you want to roll back git changes?
All right, easy. There is always a solution:
If you want to throw away all the changes on the current status and return to HEAD, write:
git reset --hard HEAD
Or, if you want to change history and leave a note that you did it, the revert
command will create a commit that reverts the changes of the commit passed to the terminal. You can use it to revert the last commit like this:
git revert <commit hash to revert>
source: git revert commit - how to undo the last commit
Tips and tricks
Make your log history prettier
Instead of git log
and scroll away the changes, use:
git log --graph --pretty=format:'%C(bold red)%an%C(reset) - %C(bold blue)%h%C(reset) - %C(bold green)(%ar)%C(reset) %C(white)%s%C(reset) %C(dim white) %C(bold cyan)%d%C(reset)'
source: this blog post.
Speaking of which…
Show git diff
with style
Install delta
and copy this into your home user ~./gitconfig
file.
also, you can see the diffs with the commits messages
with
git show
you can use shortcuts to checkout “next-to-last” commit
Instead of git checkout main
# ^ means 'first parent commit,' therefore the second-most recent commit in the main branch
git checkout main^
# If it's a merge commit, with more than one parent, this gets the second parent
git checkout main^2
# Same thing as three ^ characters - three 'first-parent' steps up the tree
git checkout main~3
# The first commit prior to a day ago
git checkout main@{yesterday}
# The first commit prior to 5 minutes ago
git checkout main@{5.minutes.ago}
source: here
Tools
You can convert markdown task lists into issues in GitHub
see source
RepoZ is a pretty nice software
https://github.com/awaescher/RepoZ
this post comes from github, view it here